Testing connectivity on a network.
DESCRIPTION
Basically, you need to have the knowledge of OSI model in networking and familiar with python language working with ICMP protocol.
Ping is an IPv4 and IPv6 testing utility that uses ICMP echo request and echo reply messages to test connectivity between hosts.
To test connectivity to another host on a network, an echo request is sent to the host address using the ping command. If the host at the specified address receives the echo request, it responds with an echo reply. As each echo reply is received, ping provides feedback on the time between when the request was sent and when the reply was received. This can be a measure of network performance.
Ping has a timeout value for the reply. If a reply is not received within the timeout, ping provides a message indicating that a response was not received. This may indicate that there is a problem, but could also indicate that security features blocking ping messages have been enabled on the network. It is common for the first ping to timeout if address resolution (ARP or ND) needs to be performed before sending the ICMP Echo Request.
After all the requests are sent, the ping utility provides a summary that includes the success rate and average round-trip time to the destination.
You can also use ping to test the ability of a host to communicate on the local network. This is generally done by pinging the IP address of the default gateway of the host. A successful ping to the default gateway indicates that the host and the router interface serving as the default gateway are both operational on the local network.
Ping can be used to test the internal configuration of IPv4 or IPv6 on the local host. To perform this test, ping the local loopback address of 127.0.0.1 for IPv4 (::1 for IPv6).
Ping can also be used to test the ability of a local host to communicate across an internetwork.
Note: Many network administrators limit or prohibit the entry of ICMP messages into the corporate network; therefore, the lack of a ping response could be due to security restrictions.
This illustrates the use of ping command.
The ping command is a very common method for troubleshooting the accessibility of devices. It uses a series of Internet Control Message Protocol (ICMP) Echo messages to determine
- Whether a remote host is active or inactive.
- The round-trip delay in communicating with the host.
- Packet loss.
The ping command first sends an echo request packet to an address, then waits for a reply. The ping is successful only if,
- the echo request gets to the destination, and
- the destination is able to get an echo reply back to the source within a predetermined time called a timeout.
The TTL value of a ping packet cannot be changed
ICMP
Although IP is only a best-effort protocol, the TCP/IP suite does provide for error messages and informational messages when communicating with another IP device. These messages are sent using the services of ICMP. The purpose of these messages is to provide feedback about issues related to the processing of IP packets under certain conditions, not to make IP reliable. ICMP messages are not required and are often not allowed within a network for security reasons.
ICMP is available for both IPv4 and IPv6. ICMPv4 is the messaging protocol for IPv4. ICMPv6 provides these same services for IPv6 but includes additional functionality. In this course, the term ICMP will be used when referring to both ICMPv4 and ICMPv6.
The types of ICMP messages, and the reasons why they are sent, are extensive. The ICMP messages common to both ICMPv4 and ICMPv6 and discussed in this module include:
- Host reachability
- Destination or Service Unreachable
- Time exceeded
Host Reachability
An ICMP Echo Message can be used to test the reachability of a host on an IP network. The local host sends an ICMP Echo Request to a host. If the host is available, the destination host responds with an Echo Reply.
Destination or Service Unreachable
When a host or gateway receives a packet that it cannot deliver, it can use an ICMP Destination Unreachable message to notify the source that the destination or service is unreachable. The message will include a code that indicates why the packet could not be delivered.
Some of the Destination Unreachable codes for ICMPv4 are as follows:
- 0 — Net unreachable
- 1 — Host unreachable
- 2 — Protocol unreachable
- 3 — Port unreachable
Some of the Destination Unreachable codes for ICMPv6 are as follows:
- 0 — No route to destination
- 1 — Communication with the destination is administratively prohibited (e.g., firewall)
- 2 — Beyond scope of the source address
- 3 — Address unreachable
- 4 — Port unreachable
Note: ICMPv6 has similar but slightly different codes for Destination Unreachable messages.
Time Exceeded
An ICMPv4 Time Exceeded message is used by a router to indicate that a packet cannot be forwarded because the Time to Live (TTL) field of the packet was decremented to 0. If a router receives a packet and decrements the TTL field in the IPv4 packet to zero, it discards the packet and sends a Time Exceeded message to the source host.
ICMPv6 also sends a Time Exceeded message if the router cannot forward an IPv6 packet because the packet has expired. Instead of the IPv4 TTL field, ICMPv6 uses the IPv6 Hop Limit field to determine if the packet has expired.
Note: Time Exceeded messages are used by the traceroute tool.
PACKAGES
import socket : socket() I used socket to capture the traffics. so created a socket connection to listen for packets .
try:
# create a socket instance
# NOTE: socket.SOCK_RAW requires sudo/root privileges
sock = socket.socket(socket.AF_INET, socket.SOCK_RAW, ICMP_CODE)
# get the host by ip
host = socket.gethostbyname(dst)
except socket.error as e:
if e.errno in ERROR_DESCR:
# Operation not permitted
print(f’{e.args[1]} {ERROR_DESCR[e.errno]}’)
else:
print(e.args[1]) # probably is the provided dst address is invalid
exit() # exit program if there is a socket error
except socket.gaierror:
return
except Exception as e:
print(e) # catch and show any other exception
return
import select : select() . It monitors sockets
import time : time() set the timeout for the socket.
time_received = time.time() # start time
# Receive the ping from the socket.
rec_packet, _ = sock.recvfrom(2048)
# end time, round to milliseconds
end = round((time.time() — time_received)*1000.0, 2)
import struct : I used struct for two ways struct.unpack() and struct.pack() for the headers.
# unpack the IP header
iph = struct.unpack(‘!BBHHHBBH4s4s’, ip_header)
# unpack the icmp header
icmph = struct.unpack(‘!BBHHH’, icmp_header)
import random : I used to return a random ID within the ICMP scope
def generate_id(timeout=2):
return int((id(timeout) * random.random()) % 65535)
And after all the debugging and using the oop the program was ready. You can find the source code in my Github.
TESTED WITH
W I N D O W S
Excute this program using WSL
- open CMD
- navigate to file path
- type the following command
python ping.py www.google.com
python ping.py 8.8.8.8
L I N U X
- open terminal
- navigate to file path
- type the following command
sudo python3 ping.py www.google.com
sudo python3 ping.py 8.8.8.8
REQUIRMENT
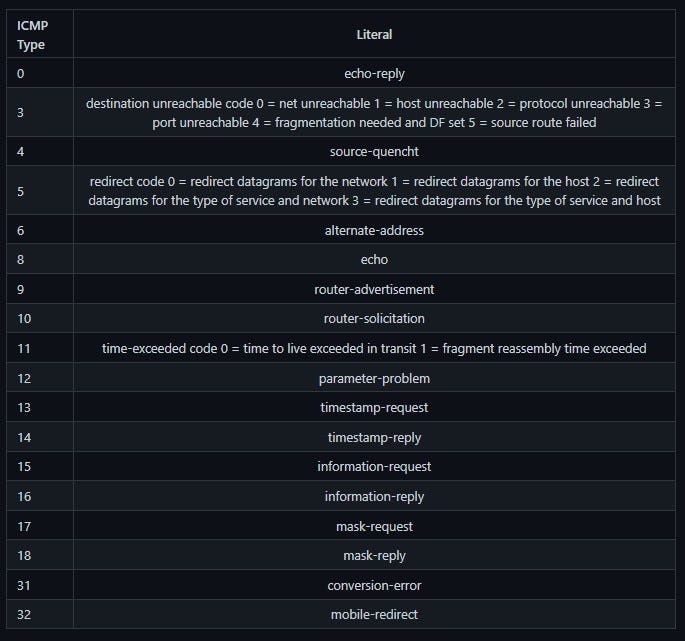
Output
- domain
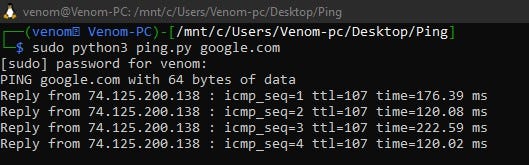
- Ip Address
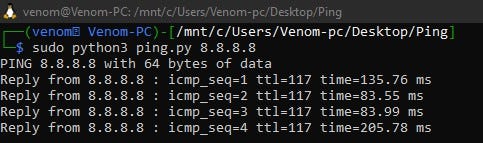
- root permission

- Help
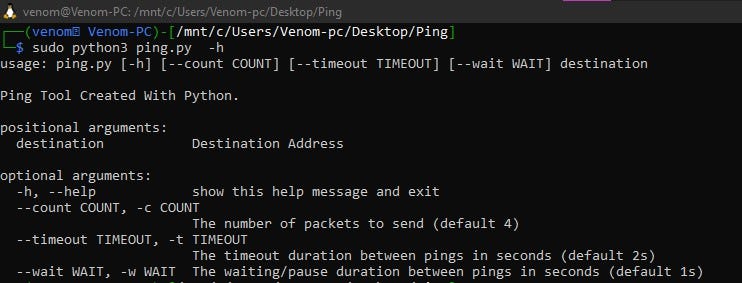
Originally published at https://github.com.