Monitor your internet traffic in real time.
DESCRIPTION
I have made my own SNIFFER TOOL using python. This illustrates the use of TCPDUMB in Unix/Linux command.
As the network grows, it becomes important to determine how to manage network traffic. It is important to understand the type of traffic that is crossing the network as well as the current traffic flow.
Tcpdump is a data-network packet analyzer computer program that runs under a command line interface. It allows the user to display TCP/IP and other packets being transmitted or received over a network to which the computer is attached
Basically, you need to understand the concept of OSI model. which means how the network works and then you can capture all the traffics.
OSI MODEL, describes seven layers that computer systems use to communicate over a network.
7 — Application : The application layer contains protocols used for process-to-process communications.
6 — Presentation : The presentation layer provides for common representation of the data transferred between application layer services.
5 — Session : The session layer provides services to the presentation layer to organize its dialogue and to manage data exchange.
4 — Transport : The transport layer defines services to segment, transfer, and reassemble the data for individual communications between the end devices.
3 — Network : The network layer provides services to exchange the individual pieces of data over the network between identified end devices.
2 — Data Link : The data link layer protocols describe methods for exchanging data frames between devices over a common media.
1 — Physical : The physical layer protocols describe the mechanical, electrical, functional, and procedural means to activate, maintain, and de-activate physical connections for a bit transmission to and from a network device.
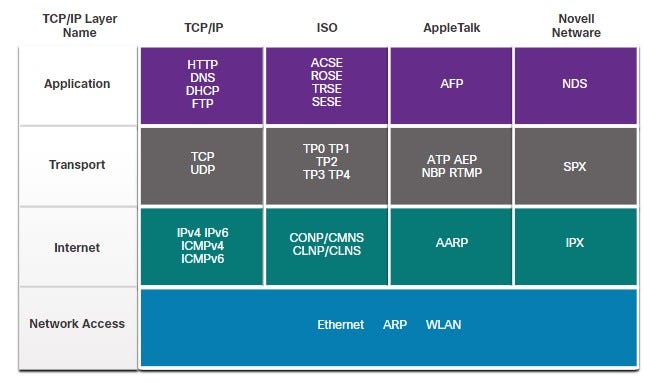
Note: Whereas the TCP/IP model layers are referred to only by name, the seven OSI model layers are more often referred to by number rather than by name. For instance, the physical layer is referred to as Layer 1 of the OSI model, data link layer is Layer2, and so on.
After all the learning, you need to spend time on Headers of protocols you want to capture. In my utility I have captured all the important protocols by using the Header details.
Headers
TCP
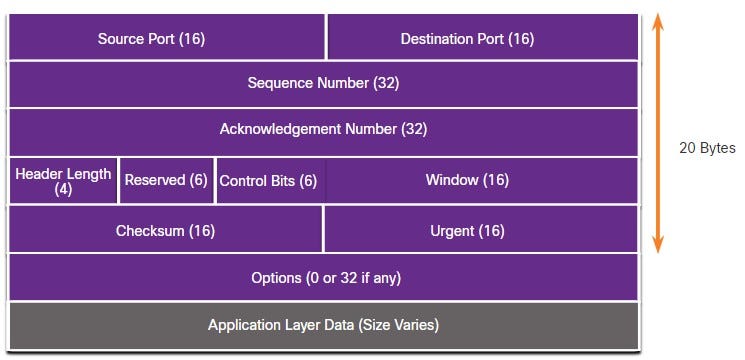
Source Port — A 16-bit field used to identify the source application by port number.
Destination Port — A 16-bit field used to identify the destination application by port number.
Sequence Number — A 32-bit field used for data reassembly purposes.
Acknowledgment Number — A 32-bit field used to indicate that data has been received and the next byte expected from the source.
Header Length — A 4-bit field known as ʺdata offsetʺ that indicates the length of the TCP segment header.
Reserved — A 6-bit field that is reserved for future use.
Control bits — A 6-bit field that includes bit codes, or flags, which indicate the purpose and function of the TCP segment.
Window size — A 16-bit field used to indicate the number of bytes that can be accepted at one time.
Checksum — A 16-bit field used for error checking of the segment header and data.
Urgent — A 16-bit field used to indicate if the contained data is urgent.
UDP
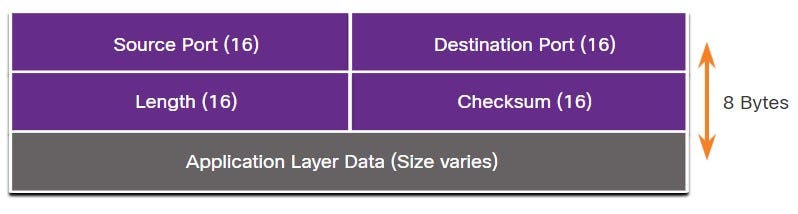
Source Port- A 16-bit field used to identify the source application by port number.
Destination Port- A 16-bit field used to identify the destination application by port number.
Length- A 16-bit field that indicates the length of the UDP datagram header.
Checksum- A 16-bit field used for error checking of the datagram header and data.
Ethernet
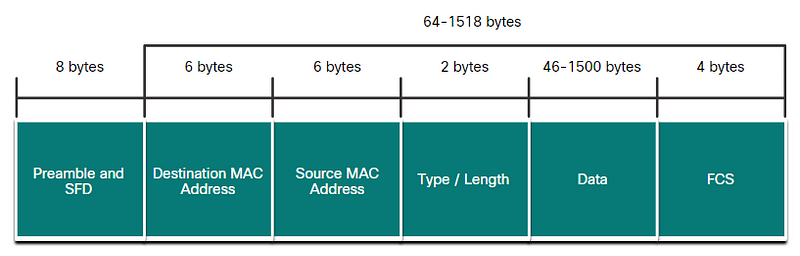
Preamble and Start Frame Delimiter Fields — The Preamble (7 bytes) and Start Frame Delimiter (SFD)
Destination MAC Address Field — This 6-byte field is the identifier for the intended recipient.
Source MAC Address Field — This 6-byte field identifies the originating NIC or interface of the frame.
Type / Length — This 2-byte field identifies the upper layer protocol encapsulated in the Ethernet frame.
Data Field — This field (46–1500 bytes) contains the encapsulated data from a higher layer.
Frame Check Sequence Field — The Frame Check Sequence (FCS) field (4 bytes) is used to detect errors in a frame.
After I understood all of these concepts, I started to code from the scratch starting with Ethernet Protocol.
I used socket to capture the traffics. so created a socket connection to listen for packets
conn = socket.socket(socket.AF_PACKET, socket.SOCK_RAW, socket.ntohs(3))
socket.ntohs(0x0003) tells capture everything including ethernet frames. To capture TCP, UDP, or ICMP only. Day by day I started to add all the headers using different Classes and it better.
PACKAGES
import socket : I used socket to capture the traffics.so created a socket connection to listen for packets .First step to analyze the Ethernet Frame and get the important information form it, and I captured it like this
# continuously receive/listen for data
raw_data, _ = conn.recvfrom(65536)
# create a new Ethernet instance with the raw_data captured
eth = Ethernet(raw_data)
dest_mac, src_mac, eth_protocol = eth.frame() # gather the frame details
# print the ethernet header info
print(f’\n — -> Ethernet Frame Destination Mac: {dest_mac}, Source Mac: {src_mac}, Type: {eth_protocol} ←-’)
After capturing the information inside the Frame, I’m analyzing it and finding out what details it provides by using this,
#Protocol Classes
class Protocol:
def __init__(self, ethernet: Ethernet):
self.data = ethernet.data # got from sniffer.py
self.eth = ethernet
# types of ethernet protocol
self.ETH_TYPE = {8:IPv4(self.data[ethernet.ETH_LEN:20+ethernet.ETH_LEN], ethernet), #0x0800
56710: IPv6(self.data[ethernet.ETH_LEN:], ethernet), #0x86dd
1544: ARP(self.data[ethernet.ETH_LEN:42]) #0x0806}
After understanding the types of ethernet protocol type, I decided to unpack the protocols by,
import struct : I used struct for two ways struct.unpack() and struct.pack() for the headers. Since this is a Sniffer tool I have to unpack the headers to get the details of the traffic on network.
# unpack the IPv6 header
self.ipv6_first_word, self.payload_legth, self.protocol, self.hoplimit = struct.unpack(“>IHBB”, data[0:8])
# unpack the IPv4 header
iph = struct.unpack(‘!BBHHHBBH4s4s’, self.ip_header)
# unpack the ICMP header
icmph = struct.unpack(‘!BBH’, icmp_header)
# unpack the TCP header
packet = struct.unpack(‘!HHLLHHHHHH’, self.eth.data[:24])
# unpack the UDP header
src_port, dest_port, size = struct.unpack(‘!HH2xH’, data[:8])
# unpack the ARP header
arph = struct.unpack(“!2s2s1s1s2s6s4s6s4s”, self.arp_header)
import binascii : To convert between binary and various ASCII-encoded binary representations. I used this package to convert the hex so I can find the details of ARP traffic.
# convert bytes to hex and then decode it as string
self.protocol_size = binascii.hexlify(arph[3]).decode(‘utf-8’)
self.protocol_type = binascii.hexlify(arph[1]).decode(‘utf-8’)
And after all the debugging and using the oop the program was ready. You can find the source code in my Github.
I have tested this program on Windows 10 Enterprise using WSL version 2.
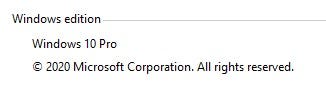
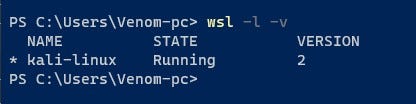
W I N D O W S
Excute this program using WSL
- open WSL Terminal
- navigate to file path
- type the following command
python sniffer.py www.google.com
python sniffer.py 8.8.8.8
L I N U X
- open terminal
- navigate to file path
- type the following command
sudo python3 sniffer.py www.google.com
sudo python3 sniffer.py 8.8.8.8
REQUIRMENT
- Run using SUDO privilege
- Run using Administration privilege
Output
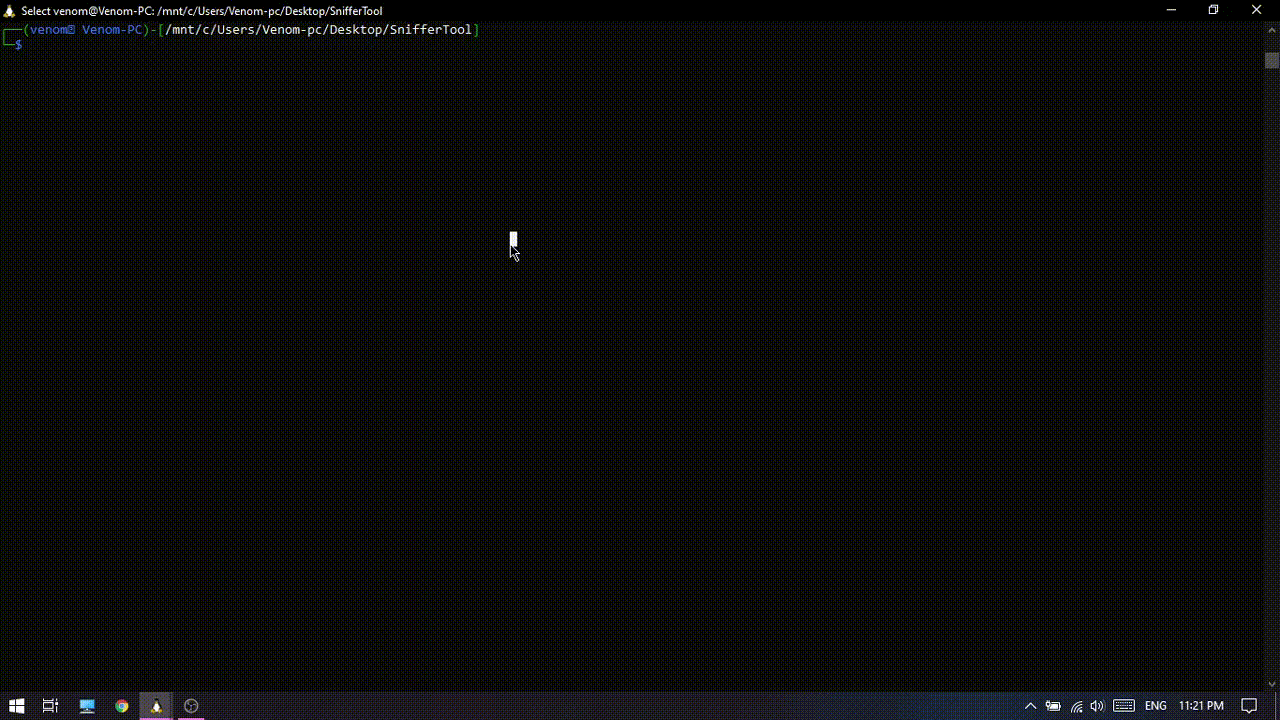
- Ethernet

- ARP
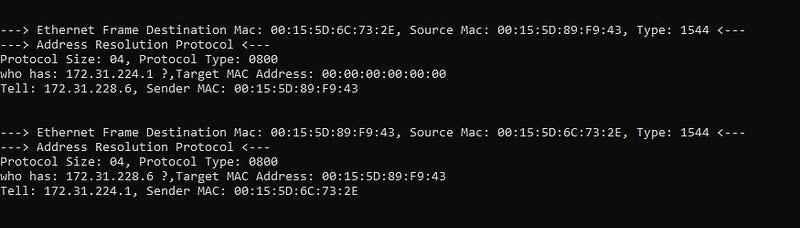
- ICMP ECHO REPLY

- ICMP ECHO REQUEST

- UDP
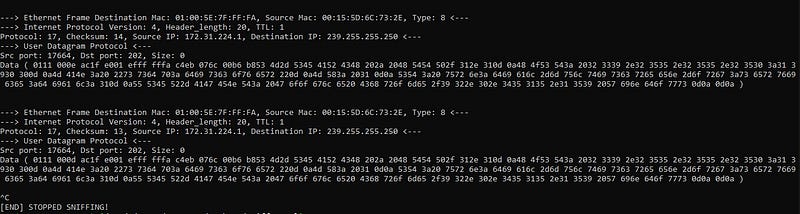
Originally published at https://github.com.